SQLite is a powerful, lightweight database engine. It is widely used because it is simple and does not require a separate server. But does it support DATETIME in DB Browser for SQLite? Let’s find out!
Understanding Data Types in SQLite
Unlike other databases like MySQL or PostgreSQL, SQLite is flexible with data types. It uses a concept called type affinity, meaning a column’s data type is more of a suggestion rather than a strict rule.
In most database systems, you have dedicated data types like DATE or DATETIME. However, SQLite handles date and time differently. Here are the most common ways to store date/time values:
- TEXT – Stores dates as strings (e.g., “2024-06-01 12:45:00”).
- INTEGER – Stores dates as Unix timestamps (seconds since 1970).
- REAL – Stores dates as Julian day numbers (floating-point values).
Each method has its pros and cons depending on your needs.
But Is There a DATETIME Type?
The short answer is no. SQLite does not have a built-in DATETIME type like other databases. But don’t worry! You can still work with date and time effectively using the text, integer, or real storage formats.
When creating a table, you can define a column as DATETIME, but SQLite will treat it as TEXT. This means that while you can store values like '2024-06-01 14:30:00'
, the database will not enforce format rules.
Using DATETIME in DB Browser for SQLite
DB Browser for SQLite is a popular tool for managing SQLite databases. But how does it handle date and time values?
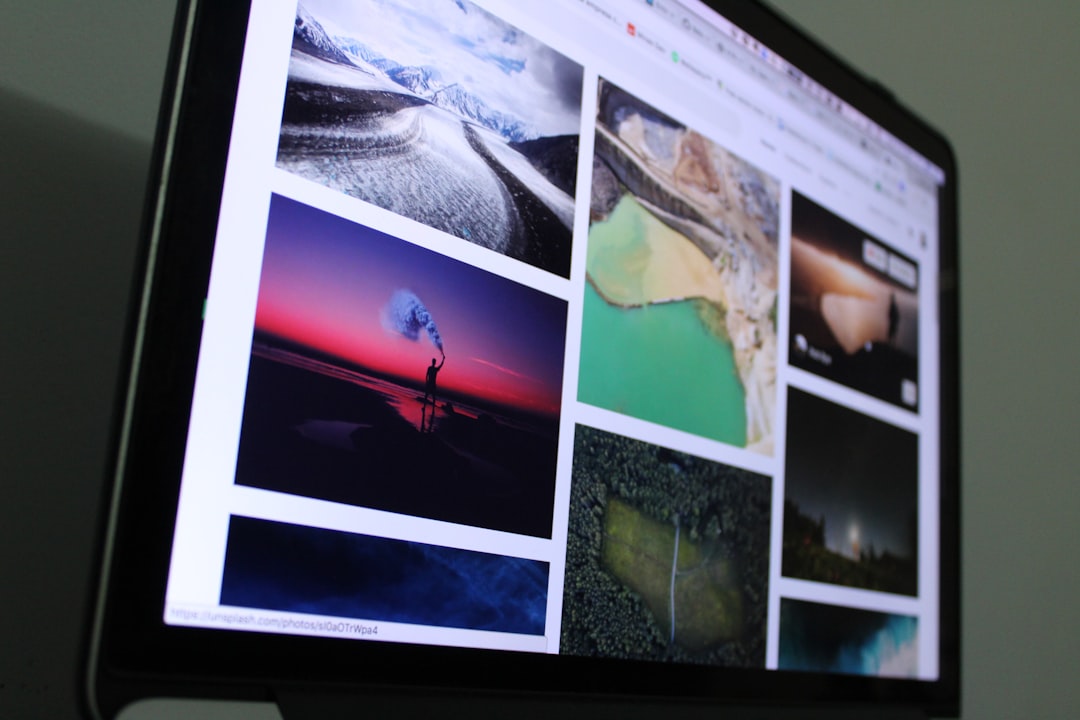
When you insert a date into a DATETIME column, it will be stored as TEXT, INTEGER, or REAL, depending on how you format it.
For example, if you run this SQL command:
CREATE TABLE events ( id INTEGER PRIMARY KEY, name TEXT, event_date DATETIME );
And insert a value using:
INSERT INTO events (name, event_date) VALUES ('Conference', '2024-06-10 09:00:00');
The DB Browser for SQLite will store '2024-06-10 09:00:00'
as a string. You can still retrieve and sort it, but there’s no built-in validation.
Working with Dates in SQLite
Even though SQLite does not enforce a strict DATETIME type, it provides useful date and time functions to work with dates.
Common Date/Time Functions
- date() – Returns a date in “YYYY-MM-DD” format.
- time() – Returns a time in “HH:MM:SS” format.
- datetime() – Returns both date and time.
- strftime() – Custom formats for date and time.
For example:
SELECT datetime('now');
This returns the current date and time like: '2024-06-01 15:45:00'
.
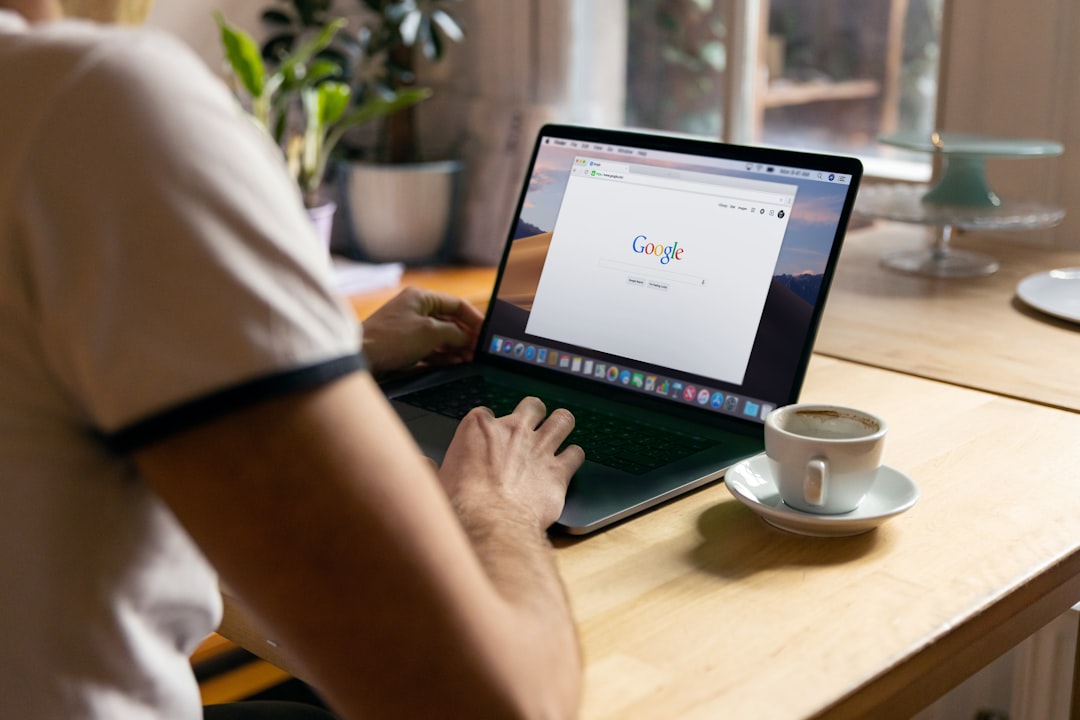
Should You Use DATETIME in SQLite?
Since SQLite does not have a strict DATETIME type, what should you do?
Recommended Approaches
- Use TEXT format for dates if you need human-readable values.
- Use INTEGER for Unix timestamps if you do a lot of date calculations.
- Use REAL for Julian day numbers if you need precision with fractional days.
If you need to ensure date validation, use application code to control the format before inserting values.
Final Thoughts
SQLite does not have a strict DATETIME type, but that doesn’t stop it from handling date and time efficiently. By using TEXT, INTEGER, or REAL and leveraging SQLite’s date functions, you can work with time data smoothly in DB Browser for SQLite.
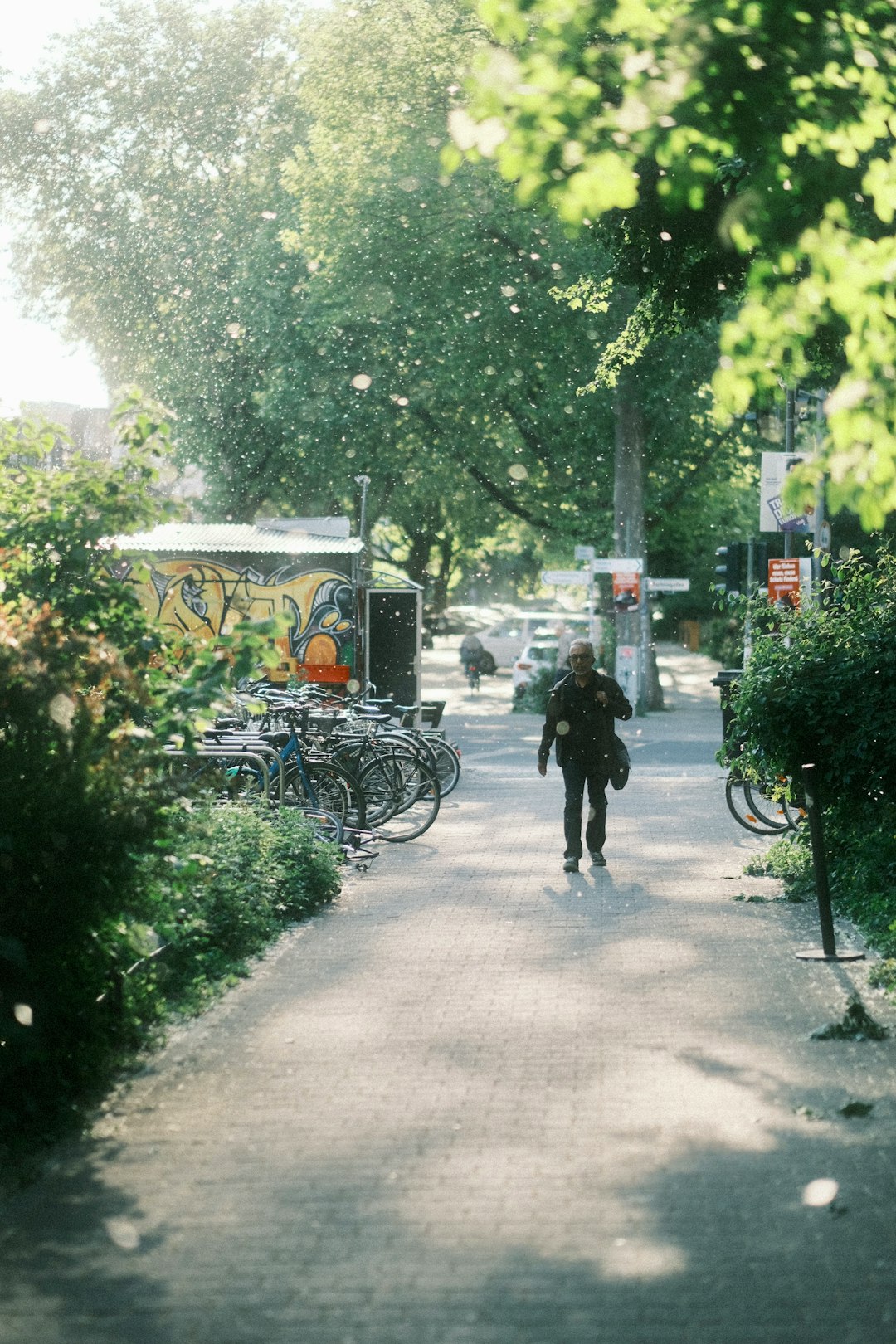
So go ahead, create your tables, and start working with dates!