If you’re working in IT or support, you’ve probably heard of Freshservice. It’s a cloud-based service desk that helps teams manage incidents, requests, and assets. But did you know you can automate Freshservice using PowerShell? That’s right — you don’t have to click around the dashboard all day!
In this guide, we’ll show you how to get started with the Freshservice PowerShell API. It’s not scary at all — just a few lines of code and some coffee.
Why Use PowerShell with Freshservice?
Here are some reasons why using PowerShell with Freshservice is super handy:
- Automate repetitive tasks like ticket creation or updates.
- Extract reports and download data quickly.
- Integrate Freshservice with other tools in your workflow.
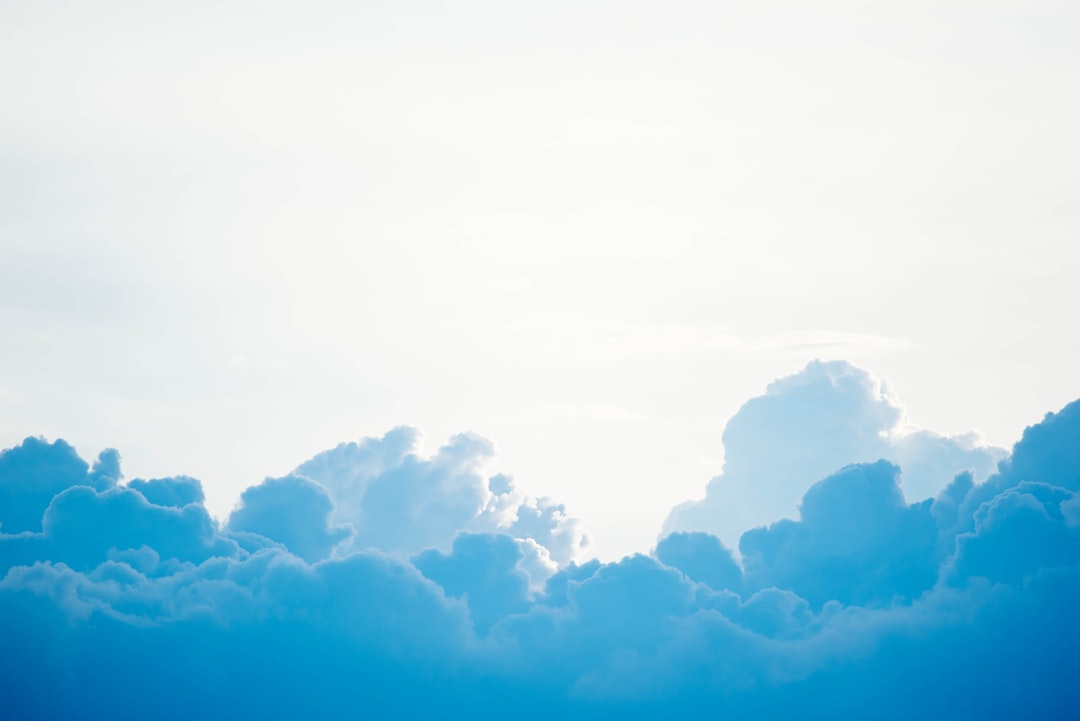
Get Ready: What You Need
Before we begin coding magic, make sure you’ve got these:
- Your Freshservice domain (It looks like yourcompany.freshservice.com).
- An API key — you can find this under your profile settings in Freshservice.
- The PowerShell console on your system.
Great! Now let’s get connected.
Step 1: Connect to the API
First, launch your PowerShell terminal. We’ll use a built-in cmdlet called Invoke-RestMethod. It allows PowerShell to send and receive data from web APIs.
$apiKey = "your_freshservice_api_key"
$domain = "yourcompany.freshservice.com"
$headers = @{
Authorization = ("Basic " + [Convert]::ToBase64String([Text.Encoding]::ASCII.GetBytes("$apiKey:X")))
}
$url = "https://$domain/api/v2/tickets"
$response = Invoke-RestMethod -Uri $url -Headers $headers -Method Get
$response
And just like that, you’ve made your first API call! If all went well, you’ll see a list of tickets pop up.
Step 2: Create a Ticket
Now let’s try something cool: creating a ticket from PowerShell.
$body = @{
description = "This is my first ticket using PowerShell!"
subject = "PowerShell API Test"
email = "user@example.com"
priority = 1
status = 2
} | ConvertTo-Json
$response = Invoke-RestMethod -Uri $url -Headers $headers -Method Post -Body $body -ContentType "application/json"
$response
There it is — your first automated ticket. Watch out Freshservice, we’re coming for your mouse clicks!
Step 3: Update or Delete a Ticket
Say you want to update a ticket. Easy peasy.
$ticketId = 12345
$url = "https://$domain/api/v2/tickets/$ticketId"
$update = @{
status = 4 # Closed
} | ConvertTo-Json
$response = Invoke-RestMethod -Uri $url -Headers $headers -Method Put -Body $update -ContentType "application/json"
$response
You can also delete a ticket. But be careful — that’s permanent!
Invoke-RestMethod -Uri $url -Headers $headers -Method Delete
Pro Tips
Here are a few tips to make your PowerShell + Freshservice life even better:
- Use Try-Catch blocks to gracefully handle failed requests.
- Explore the API docs — Freshservice has detailed guides for each endpoint.
- Schedule your scripts using Task Scheduler for daily or weekly automation.
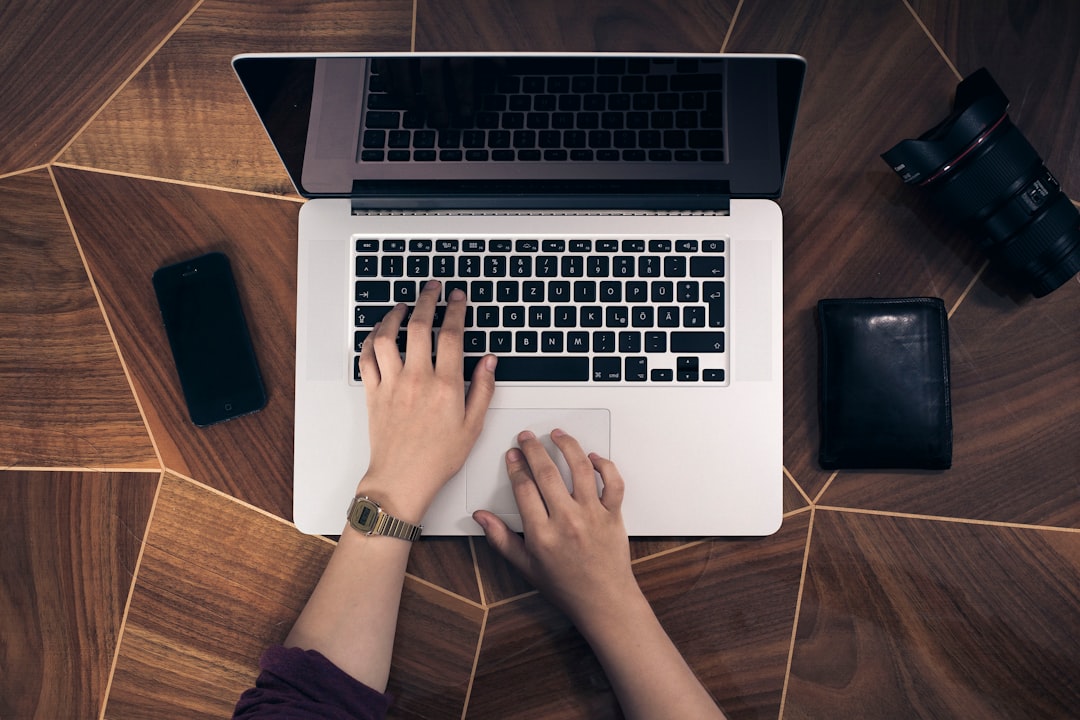
Final Thoughts
Getting started with the Freshservice PowerShell API can seem intimidating. But with a few basic commands, you’ll be scripting like a pro in no time. Whether you want to automate workflows, manage tickets faster, or just look super cool in front of your team — PowerShell has your back.
So fire up that terminal, and start building your help desk automation today. You’ve got the power (shell)!